Fillout REST API
Interact with Fillout via a REST API. Access your forms and form submissions from outside the Fillout site.
What is REST APIIntroductionAuthenticationAPI EndpointsGet formsResponseGet form metadataRequest parameters:ResponseGet all submissionsRequestResponseGet submission by idRequestResponseDelete submission by idRequestCreate a webhookRequestResponseRemove a webhookRequestResponseCreate submissionsRequestResponseRate limitsRelated articles
Using Node.js, Deno or Bun? Take a look at our JavaScript SDK: https://npmjs.com/package/@fillout/api
What is REST API
A REST API simplifies interaction with your form data, allowing for automation and integration. Following RESTful principles, it seamlessly connects to retrieve, create, and modify form data.
Introduction
The Fillout API lets you access information about your Fillout account programmatically, without using the Fillout site. The Fillout API is primarily for Fillout users and for 3rd party developers that want to build services on top of Fillout or access their submission data.
You can access a list of all your forms and the submissions for each form via the API.
Authentication
Generate and view your API key in the
Developer
settings tab of your account. You can revoke or regenerate your API key at any time via the dashboard.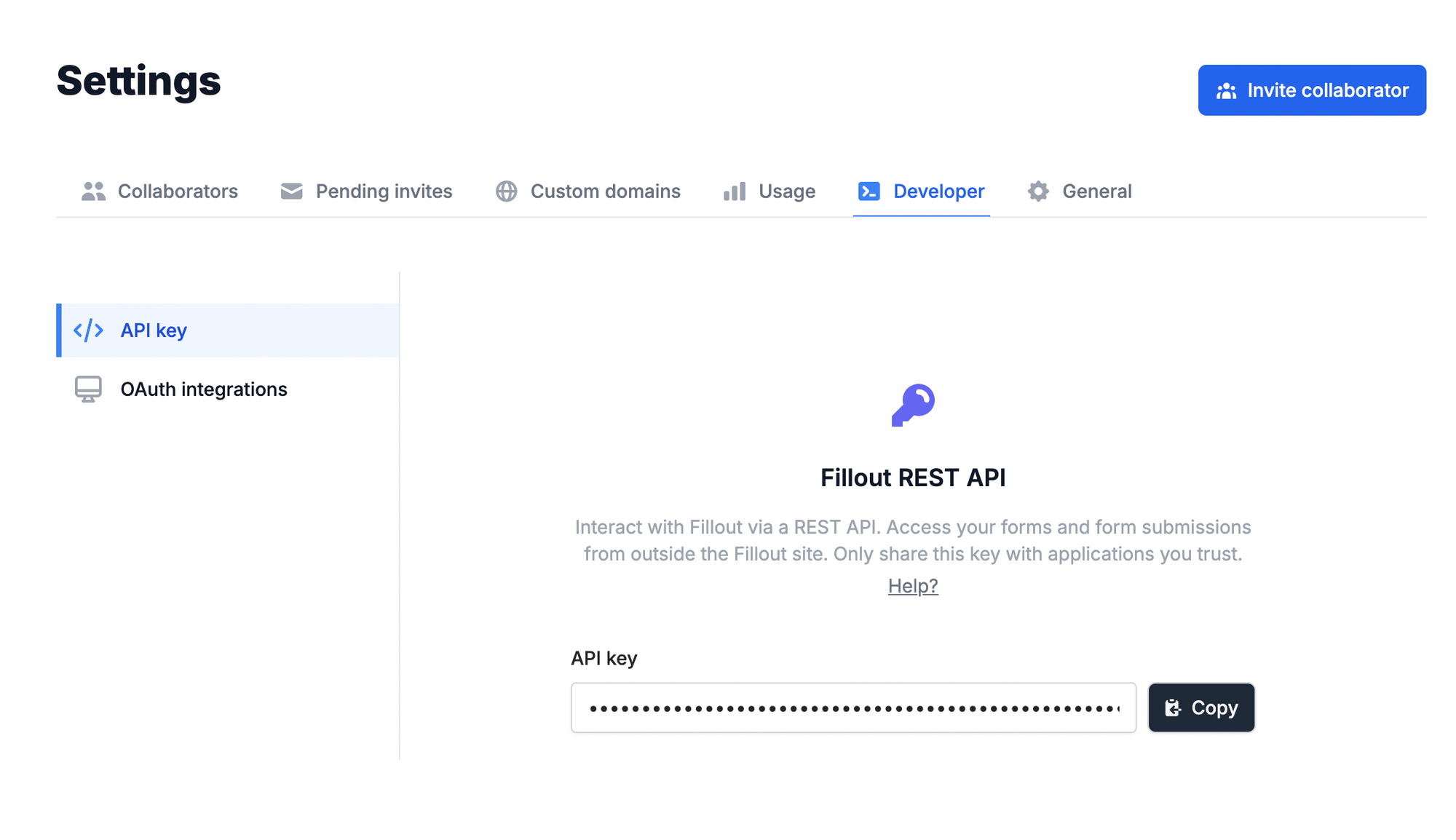
To authenticate your requests, you need to provide your API key in the
Authorization
header in the following format:Authorization: Bearer <your-api-key>
Replace
<your-api-key>
with the API key obtained from your Fillout account.You can obtain your API base URL in the API dashboard. Typically, it will be https://api.fillout.com.
Important: If you’re self-hosting Fillout or using the EU agent, a different URL will appear in the dashboard.
Note: you can also use access tokens granted via a Fillout 3rd party integration. If you’re looking to build an integration, see this article.
API Endpoints
Get forms
GET /v1/api/forms
Response
Returns a list of all your forms.
[ { "name": "My example form", "formId": "vso9PzRfHQus", }, ... ]
Get form metadata
GET /v1/api/forms/{formId}
Request parameters:
URL parameters:
formId
(the public ID of your form, you can find from/v1/api/forms
)
Response
Given the
formId
, returns all the questions in that form and other metadata.{ "id": "vso9PzRfHQus", "name": "My example form", "questions": [ { "id": "5AtgG35AAZVcrSVfRubvp1", "name": "What's your email?", "type": "Email" }, { "id": "gRBWVbE2fut2oiAMprdZpY", "name": "What is your name?", "type": "ShortAnswer" }, { "id": "hP4bHA1CgvyD2LKhBnnGHy", "name": "Pick your favorite color", "type": "MultipleChoice" } ], "calculations": [ { "id": "abcdef", "name": "Price", "type": "number" } ], "urlParameters": [ { "id": "email", "name": "email" } ], // If using Fillout Scheduling "scheduling": [ { "id": "CP4bHA1CgvyD2LKhBnnGHy", "name": "30 min meeting" } ], // If using Fillout Payments "payments": [ { "id": "Dc4bHA1CgvyD2LKhBnnCLh", "name": "Complete checkout" } ], // "quiz" only defined if quiz mode is enabled on the form "quiz": { "enabled": true, } }
The full list of possible question types:
'Address', 'AudioRecording', 'Calcom', 'Calendly', 'Captcha', 'Checkbox', 'Checkboxes', 'ColorPicker', 'CurrencyInput', 'DatePicker', 'DateRange', 'DateTimePicker', 'Dropdown', 'EmailInput', 'FileUpload', 'ImagePicker', 'LocationCoordinates', 'LongAnswer', 'Matrix', 'MultiSelect', 'MultipleChoice', 'NumberInput', 'OpinionScale', 'Password', 'Payment', 'PhoneNumber', 'Ranking', 'RecordPicker', 'ShortAnswer', 'Signature', 'Slider', 'StarRating', 'Subform', 'SubmissionPicker', 'Switch', 'Table', 'TimePicker', 'URLInput'
The full list of possible calculations:
'number', 'text', 'duration'
New field types are added regularly. Your application should discard fields with unknown types.
Get all submissions
GET /v1/api/forms/{formId}/submissions
Request
URL parameters:
formId
(required) - the public identifier of the form for which you want to retrieve submissions. This should be a string. If your form’s URL is https://form.fillout.com/t/gHretr3UKYus, the form ID is gHretr3UKYus
Query parameters:
limit
(optional) - the maximum number of submissions to retrieve per request. Must be a number between 1 and 150. Default is 50.
afterDate
(optional) - a date string to filter submissions submitted after this date
beforeDate
(optional) - a date string to filter submissions submitted before this date
offset
(optional) - the starting position from which to fetch the submissions. Default is 0.
status
(optional) - passin_progress
to get a list of in-progress (unfinished) submissions. By default, onlyfinished
submissions are returned. Note that fetching in progress submissions is available starting on the business plan.
includeEditLink
(optional) - passtrue
to include a link to edit the submission aseditLink
includePreview
(optional) - passtrue
to include preview responses
sort
(optional) - can beasc
ordesc
, defaults toasc
search
(optional) - filter for submissions containing a string of text
Date format
Both
afterDate
and beforeDate
parameters should be provided in the following format: YYYY-MM-DDTHH:mm:ss.sssZ
. For example: 2024-05-16T23:20:05.324Z. Response
{ "responses": [ { "questions": [ { "id": "abcdef", "name": "What's your name?", "type": "ShortAnswer", "value": "Timmy" } ], "calculations": [ { "id": "calculation1", "name": "price", "type": "number", "value": "12.50" } ], "urlParameters": [ { "id": "email", "name": "email", "value": "example@example.com" } ], // optional depending on if form is configured to be a quiz "quiz": { "score": 5, "maxScore": 10 }, "submissionId": "abc", "submissionTime": "2024-05-16T23:20:05.324Z", // If using Fillout Scheduling "scheduling": [ { "id": "nLJtxBJgPA", "name": "30 min meeting", "value": { "fullName": "John Smith", "email": "john@smith.com", "timezone": "Europe/London", "eventStartTime": "2024-05-20T09:00:00.000Z", "eventEndTime": "2024-05-20T09:30:00.000Z", "eventId": "du5ckkaeacd5dlj16d7ajepp8g", "eventUrl": "https://www.google.com/calendar/event?eid=ZHU1Y2trYWVhY2Q1ZGxqMTZkN2FqZXBwOGcgYXJjaGllQGZpbGxvdXQuY29t&authuser=john%40smith.com", "rescheduleOrCancelUrl": "https://schedule.fillout.com/event/3EhDXDtvEtus?t=Cq52ZAGUnDpnnlatjDKD1yjtGCFNGajY" } } ], // If using Fillout Payments "payments": [ { "id": "cLJtxCKgdL", "name": "Complete checkout", "value": { "stripeCustomerId": "cus_Ppjz3Z80000000", "stripeCustomerUrl": "https://dashboard.stripe.com/customers/cus_Ppjz3Z80000000", "stripePaymentUrl": "https://dashboard.stripe.com/payments/pi_3PRF2cFMP2ckdpfG0s0ZdJqf", "totalAmount": 1000, "currency": "USD", "email": "john@doe.com", "discountCode": "10OFF", "status": "succeeded", "paymentId": "pi_3PRF2cFMP2ckdpfG0s0ZdJqf", "stripeSubscriptionId": "sub_Ppjz3Z80000000" } } ], // If using a login pag "login": { "email": "verified@email.com" } } ], "totalResponses": 300, // total number of submissions matching given parameters "pageCount": 2 // total number of pages of submissions based on provided limit }
The question’s
type
will be a question type listed in the previous section (such as EmailInput
), while the type for a calculation is either number
or text
New field types are added regularly. Your application should discard fields with unknown types.
Get submission by id
GET /v1/api/forms/{formId}/submissions/{submissionId}
Request
URL parameters:
formId
(required) - the public identifier of the form for which you want to retrieve submissions. This should be a string. If your form’s URL is https://form.fillout.com/t/gHretr3UKYus, the form ID is gHretr3UKYus
submissionId
(required) - the identifier of the submission
Query parameters:
includeEditLink
(optional) - passtrue
to include a link to edit the submission aseditLink
Response
Same as the “Get all submissions” endpoint but only returns a single item
{ "submission": { "questions": [ { "id": "abcdef", "name": "What's your name?", "type": "ShortAnswer", "value": "Timmy" } ], "calculations": [ { "id": "calculation1", "name": "price", "type": "number", "value": "12.50" } ], "urlParameters": [ { "id": "email", "name": "email", "value": "example@example.com" } ], // If form is configued to be a quiz "quiz": { "score": 5, "maxScore": 10 }, "submissionId": "abc", "submissionTime": "2024-05-16T23:20:05.324Z", // If using Fillout Scheduling "scheduling": [ { "id": "nLJtxBJgPA", "name": "30 min meeting", "value": { "fullName": "John Smith", "email": "john@smith.com", "timezone": "Europe/London", "eventStartTime": "2024-05-20T09:00:00.000Z", "eventEndTime": "2024-05-20T09:30:00.000Z", "eventId": "du5ckkaeacd5dlj16d7ajepp8g", "eventUrl": "https://www.google.com/calendar/event?eid=ZHU1Y2trYWVhY2Q1ZGxqMTZkN2FqZXBwOGcgYXJjaGllQGZpbGxvdXQuY29t&authuser=john%40smith.com" } } ] } }
Delete submission by id
DELETE /v1/api/forms/{formId}/submissions/{submissionId}
Request
URL parameters:
formId
(required) - the public identifier of the form for which you want to retrieve submissions. This should be a string. If your form’s URL is https://form.fillout.com/t/gHretr3UKYus, the form ID is gHretr3UKYus
submissionId
(required) - the identifier of the submission
Create a webhook
POST /v1/api/webhook/create
Request
Body parameters:
formId
(required) - the public identifier of the form for which you want to retrieve submissions. This should be a string.
(required) - the endpoint where you’d like to listen for submissionsurl
Response
{ "id": 12345 }
Once subscribed, your webhook will receive submissions in the same format as the entries in the
responses
list from the /submissions
endpoint above.Remove a webhook
POST /v1/api/webhook/delete
Request
Body parameters:
webhookId
(required) - the ID of the webhook you received when you created it.
Response
N/A
Create submissions
POST /v1/api/forms/{formId}/submissions
Request
URL parameters:
formId
(required) - the public identifier of the form for which you want to import submissions. This should be a string. If your form’s URL is https://form.fillout.com/t/gHretr3UKYus, the form ID is gHretr3UKYus
Body parameters:
submissions
(required) - the maximum number of submissions that can be created in a single request is 10. The submissions to be imported should be formatted as so:
{ "submissions": [ { // required "questions": [ { "id": "abcdef", "value": "Timmy" } ], // the following are optional "urlParameters": [ { "id": "email", "name": "email", "value": "example@example.com" } ], "submissionTime": "2024-05-16T23:20:05.324Z", "lastUpdatedAt":"2024-05-17T23:20:05.324Z", "scheduling": [ { "id": "nLJtxBJgPA", "value": { "fullName": "John Smith", "email": "john@smith.com", "eventStartTime": "2024-05-20T09:00:00.000Z", "eventEndTime": "2024-05-20T09:30:00.000Z", "timezone": "Europe/London", // the following are optional "userId": 1, "scheduledUserEmail": "example@example.com" "eventId": "du5ckkaeacd5dlj16d7ajepp8g", "eventUrl": "https://www.google.com/calendar/event?eid=ZHU1Y2trYWVhY2Q1ZGxqMTZkN2FqZXBwOGcgYXJjaGllQGZpbGxvdXQuY29t&authuser=john%40smith.com", "rescheduleOrCancelUrl": "https://schedule.fillout.com/event/3EhDXDtvEtus?t=Cq52ZAGUnDpnnlatjDKD1yjtGCFNGajY" "meetingNotes": "Example meeting notes..." } } ], "payments": [ { "id": "cLJtxCKgdL", "value": { "paymentId": "pi_3PRF2cFMP2ckdpfG0s0ZdJqf", // the following are optional "stripeCustomerId": "cus_Ppjz3Z80000000", "stripeCustomerUrl": "https://dashboard.stripe.com/customers/cus_Ppjz3Z80000000", "stripePaymentUrl": "https://dashboard.stripe.com/payments/pi_3PRF2cFMP2ckdpfG0s0ZdJqf", "totalAmount": 1000, "currency": "USD", "email": "john@doe.com", "status": "succeeded", "stripeSubscriptionId": "sub_Ppjz3Z80000000" } } ], "login": { "email": "verified@email.com" } } ] }
Response
A list of created submissions formatted as described by the “Get all submissions” endpoint.
{ "submissions" : [...] }
Once imported, your submissions will be visible in the “Results” tab of the form editor.
Rate limits
All endpoints above are limited to being called no more than 5 times per second, per Account/API key.